As part of a continued commitment to a cardholder’s security, the card brands have introduced guidelines on how your shopper’s payment information can be stored and used. If you have repeat shoppers, or offer subscriptions, you must obtain your shopper's consent to store their card information for future purchases and then flag each transaction as a single purchase or as a regularly scheduled subscription.
This guide is intended to provide guidance for implementing this functionality with:
Supported cards:
- Visa
- MasterCard
- Discover
- Diners
Implementing Card on File with Payment API
If you’re using our Payment API, here are the instructions on how to implement Card on File as it relates to the storage and use of your shopper’s data.
Step 1: Add a shopper approval box using the Payment API
You must add this box to your checkout page. This is where your shoppers provide their consent to have their payment information stored for future transactions. For example:
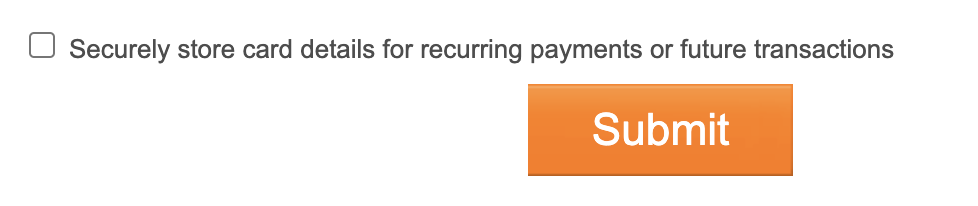
The result from this approval box is used to populate a new “store card” parameter.
Note:
If the products you sell do not require a recurring payment, or if you prefer not to store your shopper’s payment information, you can omit this approval box and set this value to “false.”
Step 2: Include parameters with your transaction requests
For the Payment API, you must send the following parameters with your transaction requests:
-
store card — Use with Auth or Auth/Capture requests to indicate if the shopper provided permissions to store their payment data. The expected value of this parameter is
true
orfalse
;true
is the default value. -
scheduled — Use with Create Merchant-Managed Subscription and Retrieve Specific Subscription to specify if the subscription is a regularly-scheduled event. The expected value of this parameter is
true
orfalse
;true
is the default value.
You might use this to have your shopper’s account automatically “topped off” when the account balance drops below a specified amount. For example, you would use "Create Merchant-Managed Subscription" withscheduled
=false
to charge the shopper's card $25 when their account balance is less than $10.
Note:
For BlueSnap-managed subscriptions and merchant-managed subscriptions, we automatically set both parameters to
true
. You only need to change the values if you want a different workflow.
Payment API Code samples
To perform a standard JSON Auth/Capture or XML Auth/Capture:
{
"amount": 11,
"storeCard": true,
"softDescriptor": "DescTest",
"cardHolderInfo": {
"firstName": "test first name",
"lastName": "test last name",
"zip": "123456"
},
"currency": "USD",
"creditCard": {
"expirationYear": "2023",
"securityCode": "837",
"expirationMonth": "02",
"cardNumber": "4263982640269299"
},
"cardTransactionType": "AUTH_CAPTURE"
}
<card-transaction xmlns="http://ws.plimus.com">
<soft-descriptor>DescTest</soft-descriptor>
<store-card>true</store-card>
<amount>11.00</amount>
<currency>USD</currency>
<card-holder-info>
<first-name>test first name</first-name>
<last-name>test last name</last-name>
<zip>123456</zip>
</card-holder-info>
<credit-card>
<card-number>4263982640269299</card-number>
<security-code>837</security-code>
<expiration-month>02</expiration-month>
<expiration-year>2023</expiration-year>
</credit-card>
</card-transaction>
To "top off" an account, use a JSON Create Merchant-Managed Subscription or XML Create Merchant-Managed Subscription:
{
"cardTransactionType": "AUTH_CAPTURE",
"transactionId": "1019988199",
"softDescriptor": "BLS*DescTest",
"amount": 11,
"usdAmount": 11,
"currency": "USD",
"cardHolderInfo": {
"firstName": "test first name",
"lastName": "test last name",
"zip": "123456"
},
"vaultedShopperId": 23902603,
"creditCard": {
"cardLastFourDigits": "9299",
"cardType": "VISA",
"cardSubType": "CREDIT",
"cardCategory": "PLATINUM",
"binCategory": "CONSUMER",
"cardRegulated": "N",
"issuingBank": "ALLIED IRISH BANKS PLC",
"issuingCountryCode": "ie"
},
"processingInfo": {
"processingStatus": "success",
"cvvResponseCode": "MA",
"avsResponseCodeZip": "U",
"avsResponseCodeAddress": "U",
"avsResponseCodeName": "U"
},
"fraudResultInfo": {
"deviceDataCollector": "N"
}
}
<?xml version="1.0" encoding="UTF-8" standalone="yes"?>
<card-transaction xmlns="http://ws.plimus.com">
<card-transaction-type>AUTH_CAPTURE</card-transaction-type>
<transaction-id>1019988369</transaction-id>
<soft-descriptor>BLS*DescTest</soft-descriptor>
<amount>11.00</amount>
<usd-amount>11.00</usd-amount>
<currency>USD</currency>
<card-holder-info>
<first-name>test first name</first-name>
<last-name>test last name</last-name>
<zip>123456</zip>
</card-holder-info>
<vaulted-shopper-id>23902609</vaulted-shopper-id>
<credit-card>
<card-last-four-digits>9299</card-last-four-digits>
<card-type>VISA</card-type>
<card-sub-type>CREDIT</card-sub-type>
<card-category>PLATINUM</card-category>
<bin-category>CONSUMER</bin-category>
<card-regulated>N</card-regulated>
<issuing-bank>ALLIED IRISH BANKS PLC</issuing-bank>
<issuing-country-code>ie</issuing-country-code>
</credit-card>
<processing-info>
<processing-status>success</processing-status>
<cvv-response-code>MA</cvv-response-code>
<avs-response-code-zip>U</avs-response-code-zip>
<avs-response-code-address>U</avs-response-code-address>
<avs-response-code-name>U</avs-response-code-name>
</processing-info>
<fraud-result-info>
<device-data-collector>N</device-data-collector>
</fraud-result-info>
</card-transaction>
Implementing Card on File with Extended API
If you’re using our Extended Payment API, here are the instructions on how to implement Card on File as it relates to the storage and use of your shopper’s data.
Step 1: Add a shopper approval box using the Extended API
You must add this box to your checkout page. This is where your shoppers provide their consent to have their payment information stored. For example:
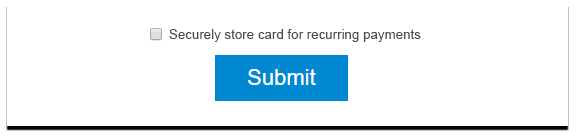
The result from this approval box are used to populate a new “store-card” parameter.
Note:
If the products you sell do not require a recurring payment, or if you prefer not to store your shopper’s payment information, you can omit this approval box and set this value to “false.”
Step 2: Include the store-card parameter with your transaction requests
For the Extended API, you must send the following parameter with your transaction requests:
- store-card– Use with Create Order and New Shopper and Create Shopping Context requests to indicate if the shopper provided permissions to store their payment data. The expected values of this parameter are “true” or “false” with the default value set as “true.”
Implementing Card on File with Hosted Solutions
If you’re using our Hosted Solutions, you notice a few changes on your checkout page.
- A checkbox lets shoppers authorize the storage of their payment information for future purchases. For example:
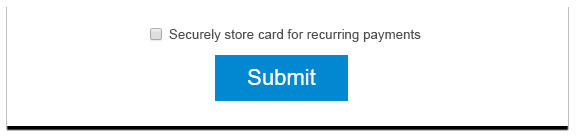
-
If the product being purchased is subscription/recurring based, this checkbox will be automatically checked. The blue button below it will say "Subscribe".
-
If the shopper is not purchasing a subscription product, the checkbox is optional and the blue button below will say "Submit". If the shopper does not check the box, we do not store the shopper’s payment information.
-
If a cart includes multiple items and there is at least one subscription product, the checkbox will automatically be checked.