Client-Side Encryption has more complex data requirements than other security solutions. As a result, BlueSnap is no longer enhancing this solution. Merchants should use Hosted Payment Fields or Secured Payment Collector to address their PCI compliance requirements.
Client-side encryption refers to encrypting sensitive data (such as the credit card number and security code) before sending it to your server. This enables you to drastically reduce your PCI compliance scope to SAQ A-EP. Client-side encryption can be easily implemented for web, Android, and iOS using a customized encryption library.
See:
- How client-side encryption works
- Implementation in your web form
- Using encrypted data to process payments using the BlueSnap APIs
How client-side encryption works
BlueSnap provides an encryption library for each platform: web, iOS, and Android. The encryption library uses your Client-Side Encryption key to encrypt sensitive payment fields such as the credit card number and security code. The encryption is done on the checkout page on the client-side device.
Encrypting payment information on the client side means that you don't have to handle sensitive data, because it is encrypted before it is sent to your server. This makes payments more secure and also reduces your PCI compliance burden.
Once you have the encrypted data on your server, you can then pass it on to BlueSnap for transaction processing using the Payment API. BlueSnap uses its private key to decrypt the data, process the transaction, and then send you information about the status of the transaction.
Implementing client-side encryption in your web form
Follow these steps in order to implement client-side encryption in your own web form:
Step 1: Add bluesnap.js
and your public encryption key
bluesnap.js
and your public encryption keyInclude the BlueSnap JavaScript library in your page, using this script:
<script src="https://gateway.bluesnap.com/js/cse/v1.0.4/bluesnap.js"></script>
The bluesnap.js script resides on the BlueSnap server.
Note:
Make sure you use the Sandbox domain when working in the Sandbox environment and the Production domain when working in the Production environment as follows:
- Sandbox URL: https://sandbox.bluesnap.com/js/cse/v1.0.4/bluesnap.js
- Production URL: https://gateway.bluesnap.com/js/cse/v1.0.4/bluesnap.js
In a separate script
tag under the first API call to create, create a new BlueSnap object and instantiate it with your public key:
<script>
var bluesnap = new BlueSnap("YOUR_PUBLIC_KEY", isSandbox);
</script>
Note:
- When working in the Sandbox environment, call: new BlueSnap("YOUR_PUBLIC_KEY", true)
- When working in the Production environment, call: new BlueSnap("YOUR_PUBLIC_KEY", false) or simply new BlueSnap("YOUR_PUBLIC_KEY") because isSandbox is set to false if not passed.
Replace YOUR_PUBLIC_KEY
with your client-side encryption key. You can find it in the BlueSnap Merchant Console under Settings > API Settings.
Note: You need a BlueSnap account in order to get your public encryption key. If you don't have an account yet, you can sign up for one here.
Step 2: Set up your form to encrypt sensitive information
Add the data-bluesnap
attribute to the card number and security code fields in your checkout form.
<div>
<label for="creditCard">Card</label>
<input type="text" placeholder="Enter Card" name="creditCard" id="creditCard" data-bluesnap="encryptedCreditCard">
</div>
<div>
<label for="cvv">CVV</label>
<input type="text" placeholder="Enter CVV" name="cvv" id="cvv" data-bluesnap="encryptedCvv">
</div>
In the same script tag where you instantiated the BlueSnap object, add code similar to the following in order to perform BlueSnap encryption once the shopper clicks the submit button:
<script>
var bluesnap = new BlueSnap
("YOUR_PUBLIC_KEY");
document.getElementById("submit-button").addEventListener("click", function(){
bluesnap.encrypt("checkout-form");
});
</script>
In bluesnap.encrypt("checkout-form")
, above, checkout-form
is the ID of the form. This tells the bluesnap.js
library where to search for input fields with the data-bluesnap
attribute.
What happens on submit?
This is what happens when the shopper clicks the submit button:
- Each of the fields with the
data-bluesnap
attribute is automatically encrypted on the client side. - An HTML hidden input field is created for each of the encrypted fields. The name of the hidden input field is the value in the
data-bluesnap
attribute. The value of the hidden field is the encrypted string. - An additional hidden field called
ccLast4Digits
is also generated. The value of this field is the last four digits of the credit card number (assuming that the credit card number field has adata-bluesnap
attribute). - The
name
attribute of the fields with thedata-bluesnap
attribute is removed, so that they are not sent to your server. - The hidden fields and the fields without the
data-bluesnap
attribute are posted to your server.
Following is an example of what would be posted to your server on submit:
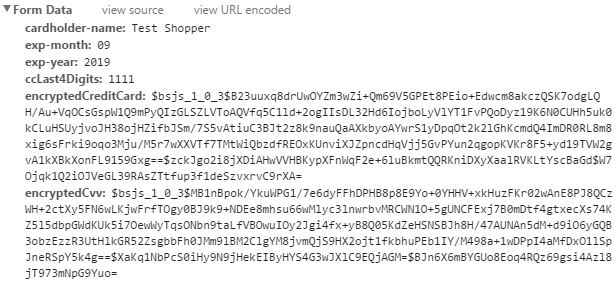
Use POST, not GET
To reinforce security measures when using Client-side Encryption, use POST, not GET.
In addition, make sure not to store any sensitive information in cookies.
Example form
For an example of a checkout form after implementing client-side encryption, see: GitHub: Checkout-page-examples/BlueSnap_CheckoutForm_CSE_Tutorial.html
Step 3: Use the encrypted information when processing payments using the API
Once you have the encrypted card details, you can process payments as usual by including those details in your API requests. See Using encrypted data to process payments using the BlueSnap APIs .
Add 3D Secure for increased checkout security
Visit the 3D Secure guide to learn how to add 3D Secure to your configuration.
Using encrypted data to process payments using the BlueSnap APIs
Once you have implemented client-side encryption, you send the encrypted card number and security code in the encryptedCardNumber
and encryptedSecurityCode
properties. These properties are within the creditCard
element in your requests. Note that you must include both of these properties in the request, or else you receive an error message.
We recommend that you test client-side encryption with the API in the Sandbox environment.
Payment API Example
Here is an example of how you can send the encrypted card details in an Auth Capture request in the Payment API.
{
"amount": 11,
"softDescriptor": "DescTest",
"cardHolderInfo": {
"firstName": "test first name",
"lastName": "test last name"
},
"currency": "USD",
"creditCard": {
"expirationYear": 2019,
"encryptedCardNumber": "$bsjs_1_0_3$B23uuxq8drUwOYZm3wZi+Qm69V5GPEt8PEio+Edwcm8akczQSK7odgLQH/Au+VqOCsGspW1Q9mPyQIzGLSZLVToAQVfq5C1ld+2ogIIsDL32Hd6IojboLyVlYT1FvPQoDyz19K6N0CUHh5uk0kCLuHSUyjvoJH38ojHZifbJSm/7S5vAtiuC3BJt2z8k9nauQaAXkbyoAYwrS1yDpqOt2k2lGhKcmdQ4ImDR0RL8m8xig6sFrki9oqo3Mju/M5r7wXXVTf7TMtWiQbzdfREOxKUnviXJZpncdHqVjj5GvPYun2qgopKVKr8F5+yd19TVW2gvA1kXBkXonFL9159Gxg==$zckJgo2i8jXDiAHwVVHBKypXFnWqF2e+6luBkmtQQRKniDXyXaalRVKLtYscBaGd$W7Ojqk1Q2iOJVeGL39RAsZTtfup3f1deSzvxrvC9rXA=",
"encryptedSecurityCode": "$bsjs_1_0_3$MB1nBpok/YkuWPG1/7e6dyFFhDPHB8p8E9Yo+0YHHV+xkHuzFKr02wAnE8PJ8QCzWH+2ctXy5FN6wLKjwFrfTOgy0BJ9k9+NDEe8mhsu66wMlyc3lnwrbvMRCWN1O+5gUNCFExj7B0mDtf4gtxecXs74KZ5l5dbpGWdKUk5i7OewWyTqsONbn9taLfVBOwuIOy2Jgi4fx+yB8Q05KdZeHSNSBJh8H/47AUNAn5dM+d9iO6yGQB3obzEzzR3UtHlkGR52ZsgbbFh0JMm9lBM2ClgYM8jvmQjS9HX2ojt1fkbhuPEb1IY/M498a+1wDPpI4aMfDxO1lSpJneRSpY5k4g==$XaKq1NbPcS0iHy9N9jHekEIByHYS4G3wJXlC9EQjAGM=$BJn6X6mBYGUo8Eoq4RQz69gsi4Azl8jT973mNpG9Yuo=",
"cardType": "VISA",
"expirationMonth": "07"
},
"cardTransactionType": "AUTH_CAPTURE"
}
Extended Payment API Example
Here is an example of how you can send the encrypted card details in a Create Order and New Shopper request in the Extended Payment API.
<batch-order xmlns="http://ws.plimus.com">
<shopper>
<web-info>
<ip>62.219.121.253</ip>
</web-info>
<shopper-info>
<shopper-currency>USD</shopper-currency>
<store-id>4677</store-id>
<locale>en</locale>
<shopper-contact-info>
<title>Mr.</title>
<first-name>John</first-name>
<last-name>Doe</last-name>
<email>[email protected]</email>
<company-name>JohnDoeAndSons</company-name>
<address1>138 Market st</address1>
<city>San Francisco</city>
<zip>756543</zip>
<state>CA</state>
<country>US</country>
<phone>1413555666</phone>
<fax>1413555666789</fax>
</shopper-contact-info>
<payment-info>
<credit-cards-info>
<credit-card-info>
<billing-contact-info>
<first-name>John</first-name>
<last-name>Doe</last-name>
<address1>138 Market st</address1>
<city>San Francisco</city>
<zip>756543</zip>
<state>CA</state>
<country>US</country>
</billing-contact-info>
<credit-card>
<encrypted-card-number>$bsjs_1_0_3$B23uuxq8drUwOYZm3wZi+Qm69V5GPEt8PEio+Edwcm8akczQSK7odgLQH/Au+VqOCsGspW1Q9mPyQIzGLSZLVToAQVfq5C1ld+2ogIIsDL32Hd6IojboLyVlYT1FvPQoDyz19K6N0CUHh5uk0kCLuHSUyjvoJH38ojHZifbJSm/7S5vAtiuC3BJt2z8k9nauQaAXkbyoAYwrS1yDpqOt2k2lGhKcmdQ4ImDR0RL8m8xig6sFrki9oqo3Mju/M5r7wXXVTf7TMtWiQbzdfREOxKUnviXJZpncdHqVjj5GvPYun2qgopKVKr8F5+yd19TVW2gvA1kXBkXonFL9159Gxg==$zckJgo2i8jXDiAHwVVHBKypXFnWqF2e+6luBkmtQQRKniDXyXaalRVKLtYscBaGd$W7Ojqk1Q2iOJVeGL39RAsZTtfup3f1deSzvxrvC9rXA=</encrypted-card-number>
<encrypted-security-code>$bsjs_1_0_3$MB1nBpok/YkuWPG1/7e6dyFFhDPHB8p8E9Yo+0YHHV+xkHuzFKr02wAnE8PJ8QCzWH+2ctXy5FN6wLKjwFrfTOgy0BJ9k9+NDEe8mhsu66wMlyc3lnwrbvMRCWN1O+5gUNCFExj7B0mDtf4gtxecXs74KZ5l5dbpGWdKUk5i7OewWyTqsONbn9taLfVBOwuIOy2Jgi4fx+yB8Q05KdZeHSNSBJh8H/47AUNAn5dM+d9iO6yGQB3obzEzzR3UtHlkGR52ZsgbbFh0JMm9lBM2ClgYM8jvmQjS9HX2ojt1fkbhuPEb1IY/M498a+1wDPpI4aMfDxO1lSpJneRSpY5k4g==$XaKq1NbPcS0iHy9N9jHekEIByHYS4G3wJXlC9EQjAGM=$BJn6X6mBYGUo8Eoq4RQz69gsi4Azl8jT973mNpG9Yuo=</encrypted-security-code>
<card-type>VISA</card-type>
<expiration-month>07</expiration-month>
<expiration-year>2019</expiration-year>
</credit-card>
</credit-card-info>
</credit-cards-info>
</payment-info>
</shopper-info>
</shopper>
<order>
<ordering-shopper>
<web-info>
<ip>62.219.121.253</ip>
<remote-host>www.merchant.com</remote-host>
<user-agent>Mozilla/5.0 (Linux; X11)</user-agent>
</web-info>
</ordering-shopper>
<cart>
<cart-item>
<sku>
<sku-id>2152762</sku-id>
</sku>
<quantity>1</quantity>
</cart-item>
</cart>
<expected-total-price>
<amount>15.00</amount>
<currency>USD</currency>
</expected-total-price>
</order>
</batch-order>
Back to Top