Learn how to implement Embedded Checkout to capture credit card payments.
If you still have questions after reading, check out the FAQs.
Deprecated
We are no longer enhancing this solution. Check out these alternative BlueSnap solutions to find one that fits your needs: New Hosted Page, Secured Payment Collector or Hosted Payment Fields.
If you want the control and flexibility offered with BlueSnap's Payment API and easily add an optimized checkout flow, Embedded Checkout is your ideal solution. Using Embedded Checkout provides your shoppers with a simple checkout flow using BlueSnap-supported currencies and in multiple languages.
This solution uses a secure form called the "Embedded Payment Form," to capture and tokenize your shopper's payment data, so sensitive data never touches your environment. When your shopper completes their purchase, you include the token in the API request to complete the transaction.
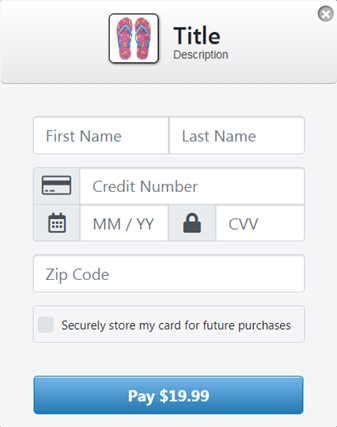
Benefits
- Easy to implement
- Securely capture payment information without your shoppers ever leaving your site
- Shoppers can checkout on any device
- Supports returning shoppers with auto-fill fields
- Merchants can configure the number of data fields, colors, logo, and description to display on the form
- Supports all BlueSnap-supported currencies
- Supports multiple languages
- Simplest level of PCI compliance: SAQ-A
Setup
For setup and usage instructions, see:
 Implementing Embedded Checkout
 Using the Embedded Checkout token to process payments
Implementing Embedded Checkout
Follow the steps below to implement Embedded Checkout.
Insert the domain for either Sandbox or Production
In all steps below, replace the BLUESNAPDOMAINPATH with the relevant domain for either the BlueSnap Sandbox or Production environment, as follows:
- Sandbox:
https://sandbox.bluesnap.com
- Production:
https://ws.bluesnap.com
For example, the Embedded Checkout token request (step 1) should be sent to
https://sandbox.bluesnap.com/services/2/payment-fields-tokens
on Sandbox andhttps://ws.bluesnap.com/services/2/payment-fields-tokens
on Production.
1. Obtain the Embedded Checkout token for the session
Obtain the Embedded Checkout token by sending a server-to-server POST request to:
BLUESNAPDOMAINPATH/services/2/payment-fields-tokens
The response provides the token in the location header. For example:
BLUESNAPDOMAINPATH/services/2/payment-fields-tokens/HOSTEDFIELDTOKENID
Notes:
- The Embedded Checkout token expires after 60 minutes.
- You need a BlueSnap account in order to generate an Embedded Checkout token. If you don't have an account yet, you can sign up for one here.
2. Add the BlueSnap JavaScript file to your checkout form
In your checkout form, call the BlueSnap Embedded Checkout JavaScript file by adding the following script:
<script type="text/javascript" src="BLUESNAPDOMAINPATH/web-sdk/4/bluesnap.js"></script>
3. Open the Embedded Checkout Form
After the script finishes loading, you can open the Embedded Payment Form. This is done in two steps:
- Set up the checkout form by calling:
bluesnap.embeddedCheckoutSetup(jsonData, callbackFunction)
- Open the checkout form by calling:
bluesnap.embeddedCheckoutOpen()
Using two separate functions lets you set up the form and it's required resources before opening the form so the shopper does not have to wait for the form to open.
Notes
- If the
bluesnap.embeddedCheckoutOpen
function is called before thebluesnap.embeddedCheckoutSetup
function, an error is displayed.- If the
bluesnap.embeddedCheckoutOpen
function is called before thebluesnap.embeddedCheckoutSetup
function has completed, the shopper sees a loading indicator.
Best Practice
Call the
bluesnap.embeddedCheckoutSetup
function when creating the token at the start of the page.
Then call thebluesnap.embeddedCheckoutOpen
function only when the shopper clicks BUY.
The bluesnap.embeddedCheckoutSetup
function has two arguments (jsonData
and callbackFunction
):
-
callbackFunction
: This argument is a callback function that is called immediately after the shopper clicks the last button ("BUY"). -
jsonData
: This JSON object lets you customize your form. It uses the following settings:
Parameter | Required/Optional | Description |
---|---|---|
token | Required | Token created by the API service |
3DS | Optional | Determines whether to apply 3DS integration. Note: The default is false. |
amount | Optional | Amount that displays in the dark blue button at the bottom of the form Note: This value must be a number. |
billingDetails | Optional | Determines whether the billing details display (uses true or false): If true, the form includes the inputted billing details: Country, State, City, Address If false, the form does not include the inputted billing details Note: The default is false. |
buttonLabel | Optional | Text that displays in the dark blue button at the bottom of the form Note: The default is "Pay." |
currency | Optional | Currency symbol that displays in the dark blue button at the bottom of the form Note: The default currency is U.S. dollars ("USD"). |
description | Optional | Description that displays below the title |
img | Optional | Relative URL path to the image uploaded to BlueSnap Note: See Uploading an image for more information. |
includeEmail | Optional | Determines whether the email address displays (uses true or false): If true, the form includes the email address If false, the form does not include the email address Note: The default is false. |
language | Optional | Embedded Payment Form's locale Note: See Available languages for more information. The default language is English ("EN"). |
shopperData | Optional | Represents the shopper's information and automatically fills in the following Embedded Checkout Form's fields: firstname lastname zip country state city address |
title | Optional | Title on the checkout form |
Note:
You cannot change the font in the Embedded Payment Form.
At this stage, you should use the HOSTEDFIELDTOKENID
to complete the transaction via an API call from your server transaction API. The callback function’s argument contains the result of the checkout form in the following format:
{
"status": "STATUS",
"code": "CODE",
"info": { // if an error or warning occurred
"errors": ["some_error"],
"warnings": ["some_warning"]
},
"cardData": {
"binCategory": "CONSUMER",
"ccBin": "400000",
"cardSubType": "CREDIT",
"ccType": "VISA",
"isRegulatedCard": "N",
"issuingCountry": "us",
"last4Digits": "1000"
},
"threeDSecure": { // if 3DS parameter was true
"authResult": "AUTH_STATUS", // example: "AUTHENTICATION_SUCCEEDED"
"threeDSecureReferenceId": "REFERENCE_ID"
}
}
The parameters are:
Parameter | Description |
---|---|
status | Result of the call. Possible values:Success Invalid Data — There is a problem with the jsonData provided from the merchant.Inner Error — There is a problem with the eCheckout .Server Error — The BlueSnap server encountered a problem. |
code | Result code. Possible values:1 — For Success 10 — For Invalid Data that prevents theeCheckout form from opening. For example: Invalid currency. 15 — For Invalid Data that does not prevent the eCheckout form from opening. For example: If the provided language is invalid, the form opens in English.20 – For Inner Error * Other codes are the standard HTTP codes. |
info | Provides the following information:errors warnings Note: The info is not included if there are no errors or warnings. |
cardData | Provides the following information:ccBin binCategory cardSubType ccType isRegulatedCard issuingCountry |
threeDSecure | Provides the following information:authResult threeDSecureReferenceId |
4. Close the BlueSnap Embedded Payment Form
The Embedded Payment Form does not close until bluesnap.embeddedCheckoutClose();
is executed. We recommend calling this function only once the transaction finishes. Refer to the example below.
var jsonData = {
token: "HOSTEDFIELDTOKENID"
title: "BlueSnap Example"
description: "This is description for example..."
img: "/developers/571747/download.jpg"
amount: "150"
currency: "EUR"
buttonLabel: "Click to buy"
billingDetails: true
includeEmail: true
language: "EN"
shopperData: {
firstname: "Someone",
lastname: "JustExample"
}
}
bluesnap.embeddedCheckoutSetup(jsonData, function (eCheckoutResult) {
if (eCheckoutResult.code == 1) { // On Success
// Your code here...
// This where you need to process the payment via an API request from your server
}
else { // On Error (code=10 - invalid jsonData, any other codes are errors, on error
if(eCheckoutResult.info){
var errors = eCheckoutResult.info.errors; // will be undefined if no errors have occurred
var warnings = eCheckoutResult.info.warnings; // will be undefined if no warnings have occurred
}
}
bluesnap.embeddedCheckoutClose();
}
Back to Top
Available languages
The following languages are available for display in the Embedded Payment Form.
Code | Language |
---|---|
BG | Bulgarian |
CZ | Czech |
DA | Danish |
DE | German |
EN | English (default language) |
ES | Spanish |
FI | Finnish |
FR | French |
HU | Hungarian |
IT | Italian |
JA | Japanese |
KR | Korean |
LT | Lithuanian |
NL | Dutch |
NO | Norwegian |
PL | Polish |
PT | Portuguese |
RU | Russian |
SK | Slovak |
SL | Slovanian |
SQ | Albanian |
SR | Serbian |
SV | Swedish |
TR | Turkish |
ZH | Chinese |
You can set the language using the language
parameter; for example, language: "EN"
sets the language as English in the following JSON example.
var jsonData = {
token: "HOSTEDFIELDTOKENID"
title: "BlueSnap Example"
description: "This is description for example..."
img: "/developers/571747/download.jpg"
amount: "150"
currency: "EUR"
buttonLabel: "Click to buy"
billingDetails: true
includeEmail: true
language: "EN"
shopperData: {
firstname: "Someone",
lastname: "JustExample"
}
}
Back to Top
Using the Embedded Checkout token to process payments
Once the callback function triggers, you can then process payments by including the Embedded Checkout token in your API requests. This must be done from your server and requires using your API Credentials.
For more information, refer to Completing Tokenized Payments.
Back to Top
Uploading an image
- In the BlueSnap Merchant Console, go to Settings > General Settings.
- Under Account Settings, click My Images.
- Click Choose File, select the image you want to upload, then click Upload.
- As mentioned above, after the file uploads you should provide the relative path of the file; for example:
- File URL:
https://sandbox.bluesnap.com/developers/571747/download.jpg
- Relative Path:
/developers/571747/download.jpg
Note:
We recommend an image of no larger than 50px by 50px to properly display inside the square. If your image is larger, we automatically resize it to fit.
If you need further assistance, contact Merchant Support.